Checkout API
The Checkout API is focused on unlocking the full potential of the payments for any or all Merchant Types. Our Checkout integration provides all the offerings Reach can provide.
The integration itself has different steps, and can vary depending on the Merchants requirements. The use-case of the Checkout API can vary, but is modular in nature, allowing Merchants to control the performance of their Payments.
Checkout features
- Local currency advantage: Access to the best wholesale FX rates
- Multiple payment methods: Supports a wide array of payment methods internationally
- Reduced card friction: Real-time card validation, number masking, and inline error messaging
- Mobile-ready: Fully responsive design
- International: Supports 36 languages
- Style customization: Customizable to suit the look of your website and Brand
- 3D Secure: Supports 3D Secure
- Fraud and compliance: Multi-factor fraud system, and PCI DSS Level 1 compliance
Integration information
Below is our Step by Step Guide to integrating the Reach Payments Solution
Before you Start
Get your environment set up for success. Below are details on your request format and how to calculate an accurate signature for requests.
1. Request Format
This contains details of the API request you will send to Reach to achieve a successful order.
Responses, formats, reference tools, and other useful information can also be found below.
Request requirements
Integration considerations
All HTTPS requests must be TLS1.2 or higher
All data is UTF-8 encoded
All field names are case sensitive
All currency and country codes must be uppercase and conform to ISO standards as specified
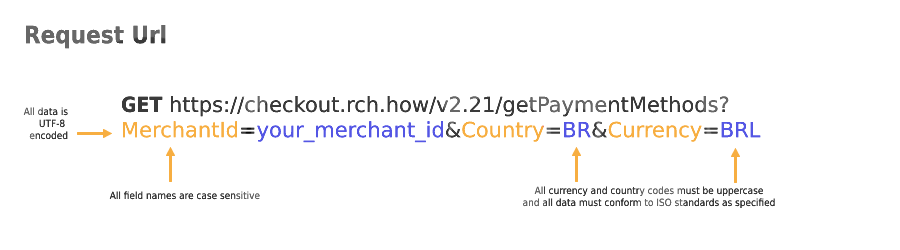
Any response parameter that is either unknown or not currently being used may safely be ignored. For example, if financing is not currently offered the financing fields in the /getPaymentMethods response may be ignored.
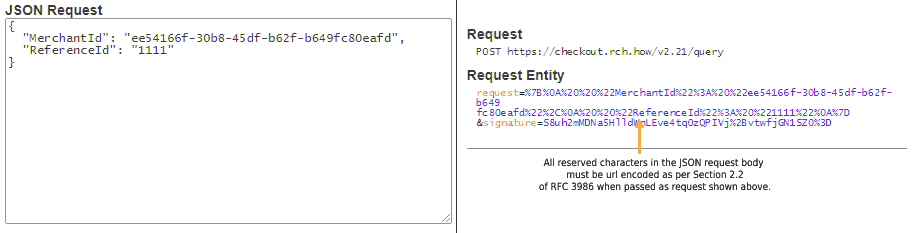
Endpoints
The following are the base URLs for accessing the production and sandbox Checkout API services.
Production: https://checkout.rch.io/
Sandbox: https://checkout.rch.how/
HTTP Format
HTTP GET
Responses to HTTP GET requests will return application/json by default.
If a Callback function is specified the JSONP pattern will be used and text/javascript will be returned to call the callback function. If the callback function does not exist a Javascript error will occur.
HTTP POST
All POST entities must be a x-www-form-urlencoded string with request and signature fields. A card field is appended when applicable.
All POST response entities will be an x-www-form-urlencoded string with response and signature fields.
Synchronous responses to the /checkout request provide important information that may be used in helping the customer have a successful order. For example, if the CardExpired error is received the customer can be redirected to the payment information page to re-enter their information and try again.
HTTP responses
Code | Definition |
---|---|
400 | Malformed request with missing or invalid required parameters |
404 | Requests with data that cannot be found in Reach's system. For instance, an unknown country code would result in a 404 response. |
503 | Temporary failure, the request should be retried |
AJAX REQUESTS
Most modern browsers support CORS but older browsers may not and so are incapable of making cross origin requests via AJAX. Support for these browsers has been deprecated. Detection of CORS support may be determined with the jQuery $.support.cors flag.
2. Signature Calculation
HMAC signatures are calculated using request or response contents and a shared secret provided by Reach. This page contains code samples demonstrating how to calculate the HMAC signature given JSON input and a shared secret string.
The general algorithm for computing a signature is as follows:
- Compute the SHA256 HMAC value of the JSON to be submitted (before any encoding) or the JSON received from Reach (after any decoding), using the shared secret provided to you by Reach.
- Base-64 encode the result.
import java.security.SignatureException;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import sun.misc.BASE64Encoder;
public class hmac_signature {
public static String compute(String json, String secret) throws SignatureException
{
try {
SecretKeySpec key = new SecretKeySpec(secret.getBytes(), DIGEST_ALGORITHM);
Mac mac = Mac.getInstance(DIGEST_ALGORITHM);
mac.init(key);
BASE64Encoder base64Encoder = new BASE64Encoder();
return base64Encoder.encode(mac.doFinal(json.getBytes("UTF-8")));
} catch (Exception e) {
throw new SignatureException("HMAC Signature failed: " + e.getMessage());
}
}
}
import hashlib
import hmac
import base64
signature = base64.b64encode(hmac.new(bytes("secret").encode('utf-8'),
bytes("json").encode('utf-8'),
digestmod=hashlib.sha256).digest())
require 'openssl'
require 'base64'
signature = Base64.strict_encode64(OpenSSL::HMAC.digest
('sha256', secret.encode('utf-8'), json.encode('utf-8')))
$signature = base64_encode(hash_hmac('sha256', $json, $secret, TRUE));
using System.Security.Cryptography;
namespace Util {
public class hmac_signature {
private string compute(string json, string secret) {
secret = secret ?? "";
var encoding = new System.Text.UTF8Encoding();
using (var hmacsha256 = new HMACSHA256(encoding.GetBytes(secret))) {
return Convert.ToBase64String(hmacsha256.ComputeHash(encoding.GetBytes(json)));
}
}
}
}
#include <openssl/hmac.h>
#include <openssl/sha.h>
void compute_signature(const unsigned char *const json,
const unsigned int json_len,
const unsigned char *const secret,
const unsigned int secret_len,
char *const signature,
unsigned int *signature_len)
{
assert(json);
assert(secret);
assert(signature);
assert(signagure_len);
static unsigned char buffer[SHA_DIGEST_LENGTH];
unsigned int len = sizeof(buffer);
HMAC(EVP_sha256(), secret, secret_length, json, json_length, buffer, &len);
base64_encode(buffer, len, signature, signature_len);
}
$ create extension pgcrypto;
$ select encode(hmac(json, secret, 'sha256'), 'base64');
//jsonFormat is the json message
//hmacSecret is your Reach hmac Secret
jsonStr=JSON.stringify(jsonFormat,null,""); //Optional depending on your IDE, jsonStr=jsonFormat might work just well
if (typeof jsonStr != 'string') {
jsonStr = JSON.stringify(jsonStr);
}
hashInBase64 = CryptoJS.enc.Base64.stringify(CryptoJS.HmacSHA256(jsonStr, hmacSecret, {asBytes: true}));
Step 1 - Get FX Rates (Optional)
To get a list of rates, you will simply send an HTTP GET request to the getRates
API. The getRates
API will then respond with either Guaranteed or Spot Rates depending on how your Reach account in configured. For more information please refer to the getRates API Specification.
Request Example
GET https://checkout.rch.how/v2.22/getRates?merchantId=yourMerchantId
GET https://checkout.rch.io/v2.22/getRates?merchantId=yourMerchantId
Further information can be found at our API endpoint here.
Step 2 - Fraud
For all transactions placed by the customer, a device fingerprint is required and is collected with the fingerprint
API request.
The information we gather with fingerprint
allows us to form a complete picture of the consumer and helps us to distinguish fraudulent behavior from normal customer behavior.
Unlike the rest of the Reach API Requests, fingerprint
needs to be loaded on the page in <script>
tags so that a pixel can be present on the frontend for our fraud system to hit and gather information about the customer's system.
Request Example
<script async="" src="https://checkout.rch.how/v2.22/fingerprint?MerchantId={SANDBOX MERCHANT ID}"></script>
<script async="" src="https://checkout.rch.io/v2.22/fingerprint?MerchantId={PROD MERCHANT ID}"></script>
Websites using Content-Security-Policy may need to include the following rules to use our fingerprint mechanism:
script-src https://checkout.rch.how/;
frame-src https://checkout.rch.how/ https://tst.kaptcha.com/
script-src https://checkout.gointerpay.net/;
frame-src https://checkout.gointerpay.net/ https://ssl.kaptcha.com/
You can find more API information on the device fingerprint here.
Step 3 - Get Payment Methods
The getPaymentMethods
API allows you to pull your specific payment methods, allowing you to show and hide dynamically the correct payment options for each currency. This will populate the options in a JSON object, in which you can display whatever best suits your website or checkout.
Request Example
<script async="" src="GET https://checkout.rch.how/v2.22/getPaymentMethods?MerchantId=6fcc882d-8aec-4f01-bb7f-a0d4be9a157b&Country=US&Currency=USD&ViaAgent=false"></script>
<script async="" src="GET https://checkout.rch.io/v2.22/getPaymentMethods?MerchantId=6fcc882d-8aec-4f01-bb7f-a0d4be9a157b&Country=US&Currency=USD&ViaAgent=false"></script>
You can find more API information on getPaymentMethods
here.
Step 4 - Badge
The Badge element should display during the checkout flow to show the customer that Reach acts as merchant of record for the transaction. Details of the Badge are returned in the badge
API call.
Request Example
https://checkout.rch.how/v2.22/badge?MerchantId=<Sandbox MerchantId>&Theme=<theme>
https://checkout.rch.how/v2.22/badge?MerchantId=2a144a21-066a-42fe-a553-736a777e39e2&Theme=light
{
"Text": "By completing your order, you will check out with Reach US, our preferred international seller, and agree to the Terms of Service of Reach US.",
"Html": "<div class=\"reach--disclosure reach--payment--banner reach--payment--banner-light\"> <div class=\"reach--logo\"> <img src=\"https://st.rch.io/logos/reach-verified-logo-grey.svg\" alt=\"Reach Verified Badge\"> </div> <div class=\"reach--content\"> <p> By completing your order, you will check out with Reach US, our preferred international seller, and agree to the <a class=\"reach--inline-link\" href=\"https://assets.rch.red/service/terms-of-service?MerchantId=18da9ea3-f9ac-4e64-8405-d301f079a658\" title=\"Read Terms of Service\" target=\"reach\">Terms of Service</a> of <strong>Reach US</strong>. </p> </div> </div>",
"ImageUrl": "https://st.rch.io/logos/reach-verified-logo-grey.svg",
"FlagImageUrl": "https://st.rch.io/flags/US.png",
"TermsOfServiceUrl": "https://assets.rch.red/service/terms-of-service?MerchantId=18da9ea3-f9ac-4e64-8405-d301f079a658",
"MerchantOfRecord": "Reach US",
"MerchantOfRecordUrl": "https://withreach.com",
"MerchantName": "Jenna's Capes"
}
The theme of the badge is specified by the Theme parameter. Valid values for theme are: light or dark. If the Theme parameter is not specified, the request will default to the light theme.
The location declaration badge must be displayed prior to the final confirmation of the transaction by the customer. The badge may be displayed on the final order confirmation screen or in the pages leading up to final confirmation page.
Be advised that a link to a separate webpage is not accepted by the schemes; location information must be disclosed within the checkout.
Render Banner and Badge Server-Side
An IP address may also be added to the badge
request to generate the Badge on the server and serve the result to your client. The badge
response will be based on the IP specified rather than the IP associated with the request.
Request Example
https://checkout.rch.how/v2.22/badge?MerchantId=<MerchantId>&Theme=<theme>&ConsumerIpAddress=<ip_address>
https://checkout.rch.how/v2.22/badge?MerchantId=2a144a21-066a-42fe-a553-736a777e39e2&Theme=dark&ConsumerIpAddress=153.128.10.226
{
"Text": "By completing your order, you will check out with Reach US, our preferred international seller, and agree to the Terms of Service of Reach US.",
"Html": "<div class=\"reach--disclosure reach--payment--banner reach--payment--banner-dark\"> <div class=\"reach--logo\"> <img src=\"https://st.rch.io/logos/reach-verified-logo-white.svg\" alt=\"Reach Verified Badge\"> </div> <div class=\"reach--content\"> <p> By completing your order, you will check out with Reach US, our preferred international seller, and agree to the <a class=\"reach--inline-link\" href=\"https://assets.rch.red/service/terms-of-service?MerchantId=18da9ea3-f9ac-4e64-8405-d301f079a658\" title=\"Read Terms of Service\" target=\"reach\">Terms of Service</a> of <strong>Reach US</strong>. </p> </div> </div>",
"ImageUrl": "https://st.rch.io/logos/reach-verified-logo-white.svg",
"FlagImageUrl": "https://st.rch.io/flags/US.png",
"TermsOfServiceUrl": "https://assets.rch.red/service/terms-of-service?MerchantId=18da9ea3-f9ac-4e64-8405-d301f079a658",
"MerchantOfRecord": "Reach US",
"MerchantOfRecordUrl": "https://withreach.com",
"MerchantName": "Jenna's Capes"
}
For further API information on the Badge, please visit our documentation here.
details
Step 5 - Checkout
This is where the magic happens! The checkout
call allows a merchant to POST data to our checkout
endpoint, in hopes of returning a successful payment response. What Reach will do with this information is a lot different from other processors. Where it differs is in its payment model or Merchant of Record (MOR).
Request Example
POST https://checkout.rch.how/v2.22/checkout
"request": {
"MerchantId": "x",
"ReferenceId": "112233",
"PaymentMethod": "MC",
"ConsumerTotal": "63.91",
"ConsumerCurrency": "CAD",
"RateOfferId": "ee9efd25-e27a-4359-9312-aff3e3869b48",
"Capture": true,
"ViaAgent": true,
"AcceptLiability": true,
"OpenContract": false,
"DeviceFingerprint": "xyx",
"ContractId": "b229209b-9658-4538-b326-563eeebfaa5b",
"Items": [
{
"Sku": "Item1",
"ConsumerPrice": "63.91",
"Quantity": "1"
}
],
"Consumer": {
"Name": "john smith",
"Email": "[email protected]",
"Address": "394 Stanhope Garden",
"City": "Winnipeg",
"Region": "MB",
"PostalCode": "R2N 0G8",
"Country": "CA"
}
}
POST https://checkout.rch.io/v2.22/checkout
"request": {
"MerchantId": "x",
"ReferenceId": "112233",
"PaymentMethod": "MC",
"ConsumerTotal": "63.91",
"ConsumerCurrency": "CAD",
"RateOfferId": "ee9efd25-e27a-4359-9312-aff3e3869b48",
"Capture": true,
"ViaAgent": true,
"AcceptLiability": true,
"OpenContract": false,
"DeviceFingerprint": "xyx"
"ContractId": "b229209b-9658-4538-b326-563eeebfaa5b",
"Items": [
{
"Sku": "Item1",
"ConsumerPrice": "63.91",
"Quantity": "1"
}
],
"Consumer": {
"Name": "john smith",
"Email": "[email protected]",
"Address": "394 Stanhope Garden",
"City": "Winnipeg",
"Region": "MB",
"PostalCode": "R2N 0G8",
"Country": "CA"
}
}
For further API details and specifications, please visit our API reference material here.
Step 6 - Capture
For Merchants or Partners who require delayed payment capture on orders, it is required to capture the order within 7 days of Authorization. To delay capture, your checkout
call above must already set the Parameter of AutoCapture=false
. Once you are shipping the order or capturing the order, the API call must happen similar to as follows:
Request Example
POST https://checkout.rch.how/v2.22/capture
{
"request":
{
"MerchantId":
"6f123-10erofogl-1212359",
"OrderId":
"12345"
},
"signature":
"abcdef="
}
POST https://checkout.rch.io/v2.22/capture
{
"request":
{
"MerchantId":
"6f123-10erofogl-1212359",
"OrderId":
"12345"
},
"signature":
"abcdef="
}
For further details on Capturing an Order please visit our API guide here. Along with this, you can review the Capture rules in our Checkout documentation here.
Step 7 - Refund
Refunds are available in your Reach Admin, but you can also use our endpoint to execute refunds against your orders. For more information click here.
Step 8 - List Reports (Optional)
List Reports are used for accounting purposes, and will be available at our endpoint when remittance has been completed. You can find the API information here.
Order states
The below information provides a brief overview of all order, refund, contract, and review states that a merchant will experience.
Order States
Pending | Transient state while the order is waiting for a payment attempt to be made. |
Processing* | A payment attempt requiring external authentication (e.g. PayPal) is in progress. |
PaymentAuthorized* | Payment has been authorized. If the order is not under review payment capture may proceed. |
Processed* | Payment for the order has completed successfully. |
ProcessingFailed* | The payment attempt for the order was not successful. |
Cancelled* | The order was cancelled explicitly by the merchant or the order expired before being captured. |
Declined* | The order was declined as a result of a manual fraud review. |
*Asynchronous notifications are sent when these states are reached.
Order state diagram
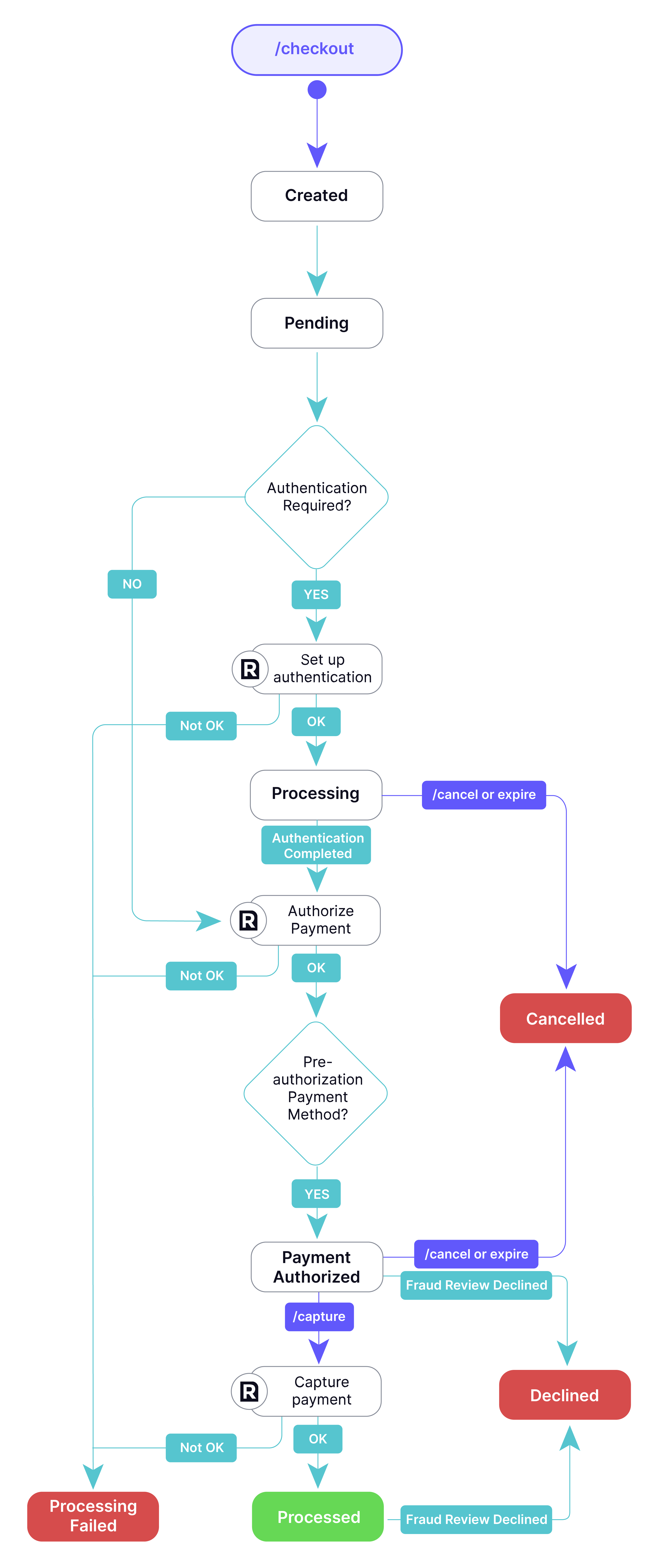
Refund States
Refund states are independent of the state of the order the refund is associated with.
Attempting to process a refund again after an initial failure may or may not be successful. Reach personnel are notified and investigate each refund failure.
InProgress | The refund is still pending. |
Succeeded* | The refund succeeded. |
Failed* | The refund failed. |
*Asynchronous notifications are sent when these states are reached.
Refund state diagram
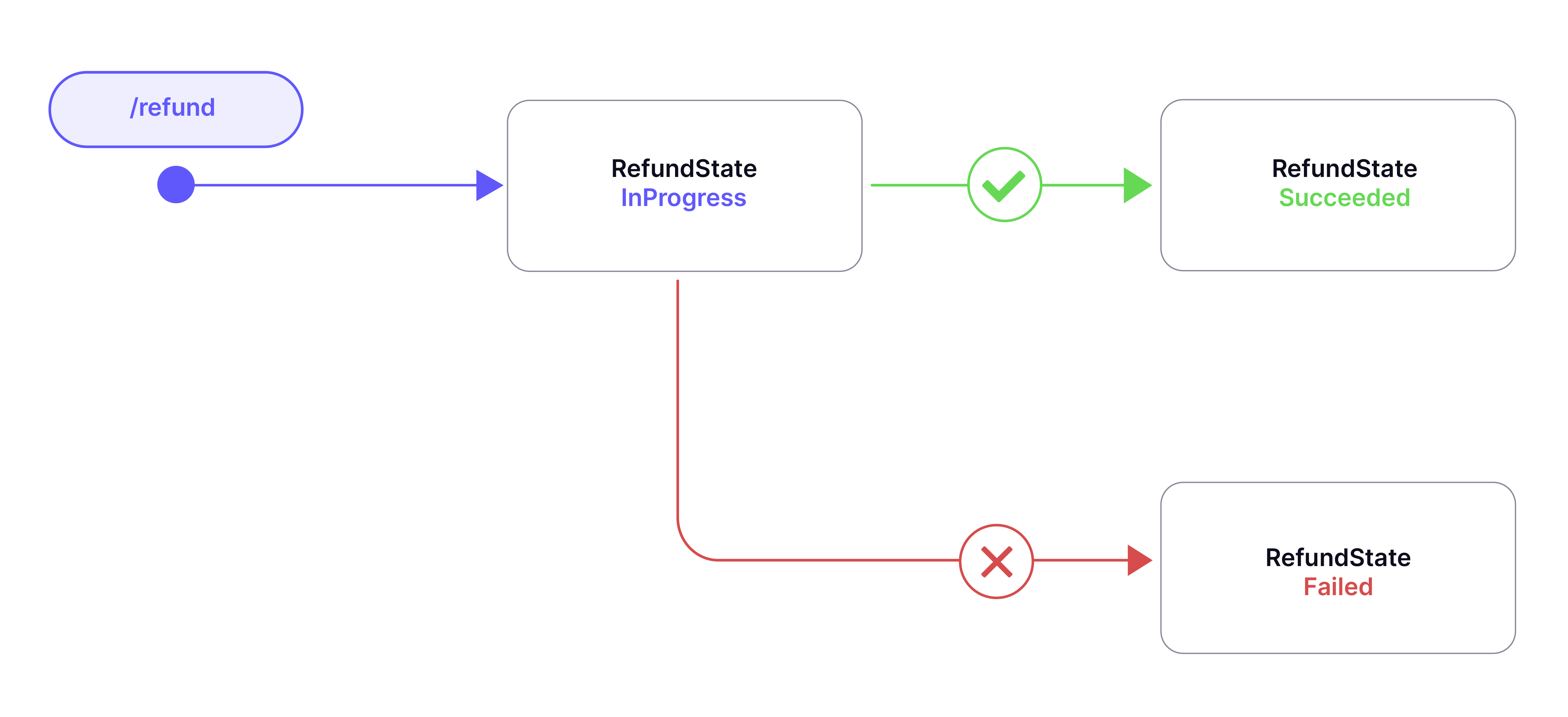
Fraud States
Fraud reviews
Review states are independent of the state of the order being reviewed. Reviews are initiated and resolved by Reach's fraud team.
Under review states
True | The payment attempt is under review. |
False | The payment attempt is not under review. |
Notification events
Order Under Review | The payment attempt is under review. Fulfillment should not continue regardless of order state. |
Order Approved | The payment attempt has been approved and is no longer under review. Fulfillment may continue if the order is in a completed state. |
Order Declined | The payment attempt has been declined and the order state has been set to Declined. Fulfillment should not continue. |
Fraud state diagram
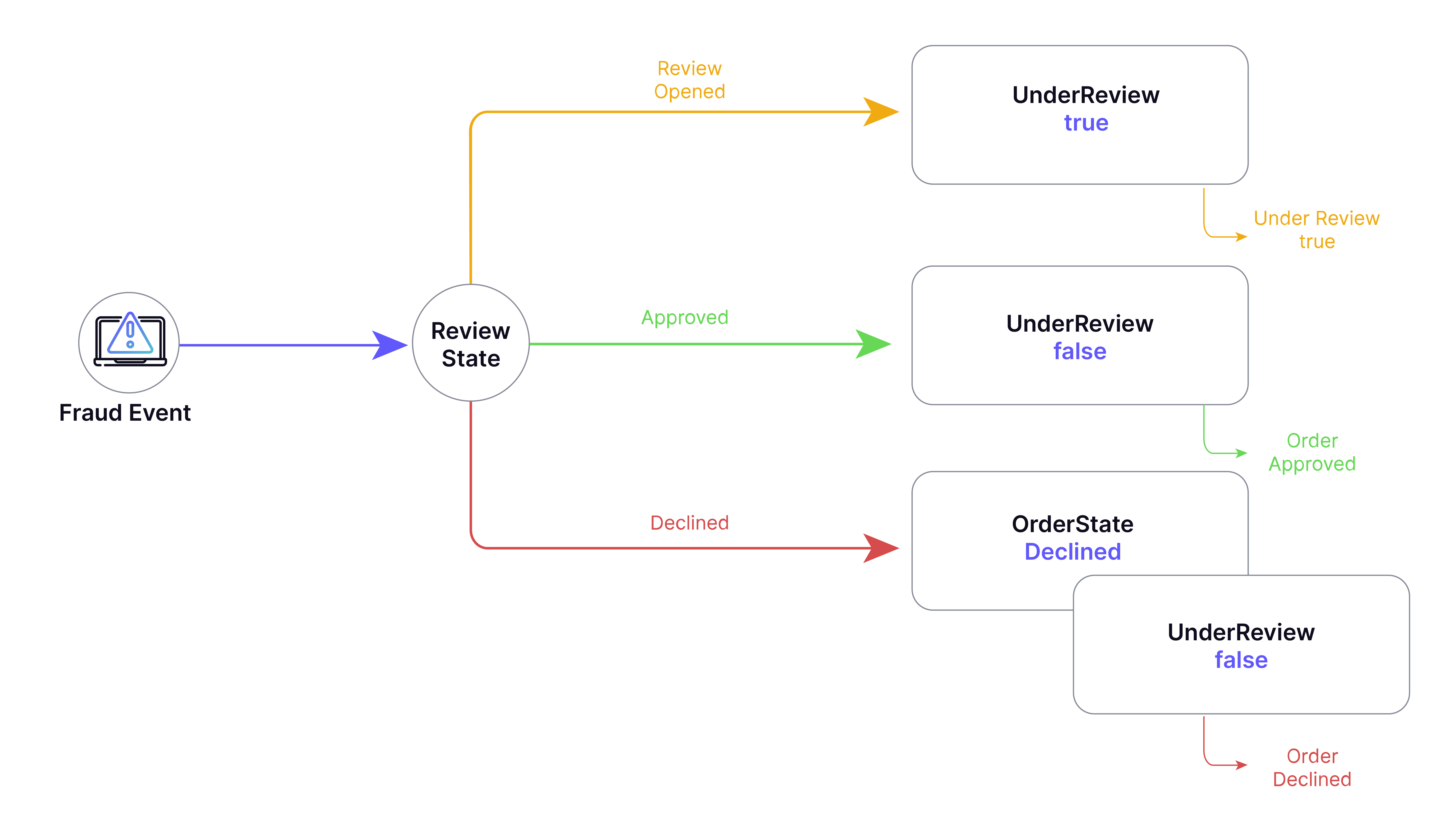
Contract States
Open* | The contract is open and the associated ContractId may be used in subsequent /create, /checkout, and /authorize requests. |
Closed* | The contract is closed and the ContractId may not be used for new orders. |
*Asynchronous notifications are sent when these states are reached.
Contract state diagram
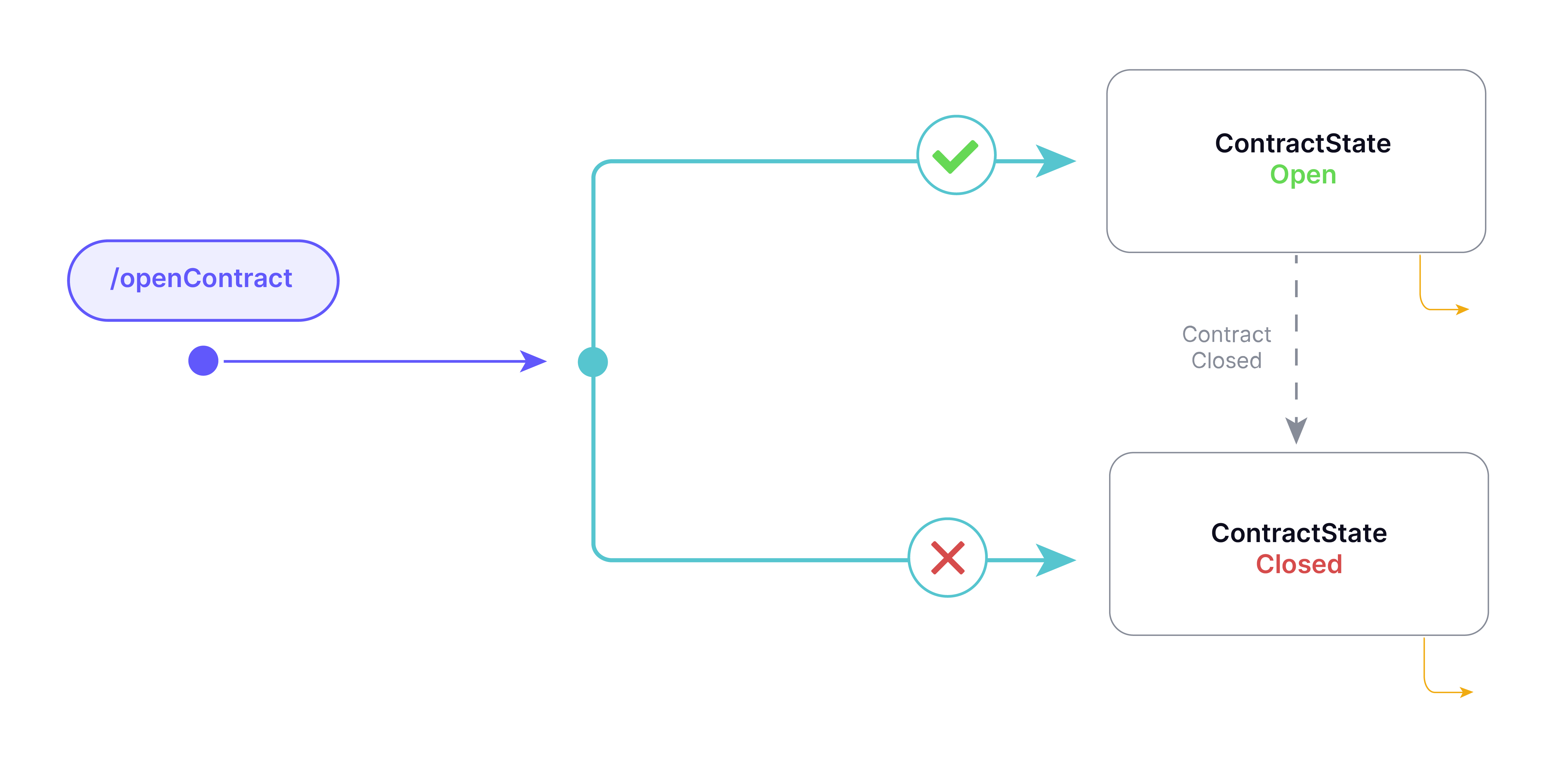
Notifications
Notification events are sent to merchants via asynchronous HTTP POST requests in order to synchronize the merchant's payment information with Reach's information. Technical information around notification structure can be found in the API Notifications Guide.
Updated 3 months ago